如图片所示
这里采用方案是:
1,使用jbarcode生成一维条码。(这里需要注意一点,jbc.setCheckDigit(false)不需要验校验位,不然会出现数据长度问题)
2,使用java程序生成上半部信息。
3,将图片进行拼接。
以下是代码:
class="java" name="code">package com.duduli.li;
import java.awt.Color;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.font.FontRenderContext;
import java.awt.geom.Rectangle2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.List;
import javax.imageio.ImageIO;
import org.jbarcode.JBarcode;
import org.jbarcode.JBarcodeFactory;
import org.jbarcode.encode.EAN13Encoder;
import org.jbarcode.encode.InvalidAtributeException;
import org.jbarcode.paint.TextPainter;
import org.jbarcode.util.ImageUtil;
public class BarcodeImageWithText {
// 1,生成一维条码。
private static final int BARCODE_HEIGHT = 20;
private static final int BARCODE_DPI = ImageUtil.DEFAULT_DPI;
private static final String FONT_FAMILY = "console";
private static final int FONT_SIZE = 14;
private static JBarcode jbc = null;
static JBarcode getJBarcode() throws InvalidAtributeException {
if (jbc == null) {
jbc = JBarcodeFactory.getInstance().createEAN13();
jbc.setEncoder(EAN13Encoder.getInstance());
jbc.setTextPainter(CustomTextPainter.getInstance());
jbc.setBarHeight(BARCODE_HEIGHT);
jbc.setXDimension(Double.valueOf(0.4).doubleValue());
jbc.setCheckDigit(false);// 对于固定条码的时候这里在条码中加入校验位,导致你写入13位提示14位
jbc.setShowText(true);
}
return jbc;
}
public static void createBarcode(String message) {
try {
File file = new File("d:/" + message + ".png");
FileOutputStream fos = new FileOutputStream(file);
createBarcode(message, fos);
fos.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static void createBarcode(String message, OutputStream os) {
try {
BufferedImage image = getJBarcode().createBarcode(message);
ImageUtil.encodeAndWrite(image, ImageUtil.PNG, os, BARCODE_DPI, BARCODE_DPI);
os.flush();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
protected static class CustomTextPainter implements TextPainter {
private static CustomTextPainter instance = new CustomTextPainter();
public static CustomTextPainter getInstance() {
return instance;
}
public void paintText(BufferedImage barCodeImage, String text, int width) {
Graphics g2d = barCodeImage.getGraphics();
Font font = new Font(FONT_FAMILY, Font.PLAIN, FONT_SIZE * width);
g2d.setFont(font);
FontMetrics fm = g2d.getFontMetrics();
int height = fm.getHeight();
int center = (barCodeImage.getWidth() - fm.stringWidth(text)) / 2;
g2d.setColor(Color.WHITE);
g2d.fillRect(0, 0, barCodeImage.getWidth(), barCodeImage.getHeight() * 1 / 20);
g2d.fillRect(0, barCodeImage.getHeight() - (height * 9 / 10), barCodeImage.getWidth(), (height * 9 / 10));
g2d.setColor(Color.BLACK);
g2d.drawString(text, center, barCodeImage.getHeight() - (height / 10) - 2);
}
}
// 2, 生成头部的商品信息
public static void exportImg(List<String> list) {
int width = 174;
int height = 76;
File file = new File("d:/image.png");
Font font = new Font("console", Font.BOLD, 14);
BufferedImage bi = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics2D g2 = (Graphics2D) bi.getGraphics();
g2.setBackground(Color.WHITE);
g2.clearRect(0, 0, width, height);
g2.setPaint(Color.BLACK);
for (int i = 0; i < list.size(); i++) {
FontRenderContext context = g2.getFontRenderContext();
Rectangle2D bounds = font.getStringBounds(list.get(i), context);
double x = (width - bounds.getWidth()) / 2;
double y = (height - bounds.getHeight()) / 2;
double ascent = -bounds.getY();
double baseY = y + (ascent * (i - 1));
g2.drawString(list.get(i), (int) x, (int) baseY);
}
try {
ImageIO.write(bi, "png", file);
} catch (IOException e) {
e.printStackTrace();
}
}
// 3,合并图片
public static boolean mergeImage(String[] imgs, String type, String dst_pic) {
int len = imgs.length;
if (len < 1) {
return false;
}
File[] src = new File[len];
BufferedImage[] images = new BufferedImage[len];
int[][] ImageArrays = new int[len][];
for (int i = 0; i < len; i++) {
try {
src[i] = new File(imgs[i]);
images[i] = ImageIO.read(src[i]);
} catch (Exception e) {
e.printStackTrace();
return false;
}
int width = images[i].getWidth();
int height = images[i].getHeight();
ImageArrays[i] = new int[width * height];
ImageArrays[i] = images[i].getRGB(0, 0, width, height, ImageArrays[i], 0, width);
}
int dst_height = 0;
int dst_width = images[0].getWidth();
for (int i = 0; i < images.length; i++) {
dst_width = dst_width > images[i].getWidth() ? dst_width : images[i].getWidth();
dst_height += images[i].getHeight();
}
if (dst_height < 1) {
return false;
}
try {
BufferedImage ImageNew = new BufferedImage(dst_width, dst_height, BufferedImage.TYPE_INT_RGB);
int height_i = 0;
for (int i = 0; i < images.length; i++) {
ImageNew.setRGB(0, height_i, dst_width, images[i].getHeight(), ImageArrays[i], 0, dst_width);
height_i += images[i].getHeight();
}
File outFile = new File(dst_pic);
ImageIO.write(ImageNew, type, outFile);// 写图片 ,输出到硬盘
} catch (Exception e) {
e.printStackTrace();
return false;
}
return true;
}
public static void main(String[] args) {
List<String> list = new ArrayList<String>();
list.add("商品类型:手机");
list.add("品牌:魅族");
list.add("商品价格:3199");
list.add("有效时间:2019-6-30");
exportImg(list);
String code = "6974323434245";
createBarcode(code);
String s[] = { "d:/image.png", "d:/" + code + ".png" };
mergeImage(s, "png", "d:/code/code_" + code + ".png");
for (String str : s) {
new File(str).delete();
}
}
}
- commons-lang3-3.8.jar (490.1 KB)
- 下载次数: 0
- jbarcode-0.2.8.jar (58.6 KB)
- 下载次数: 0
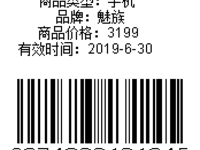
- 大小: 1.4 KB